AppBar with Title |
AppBar(
backgroundColor: Colors.red,
title: new Text("Title",),
elevation: 4.0,
),
| 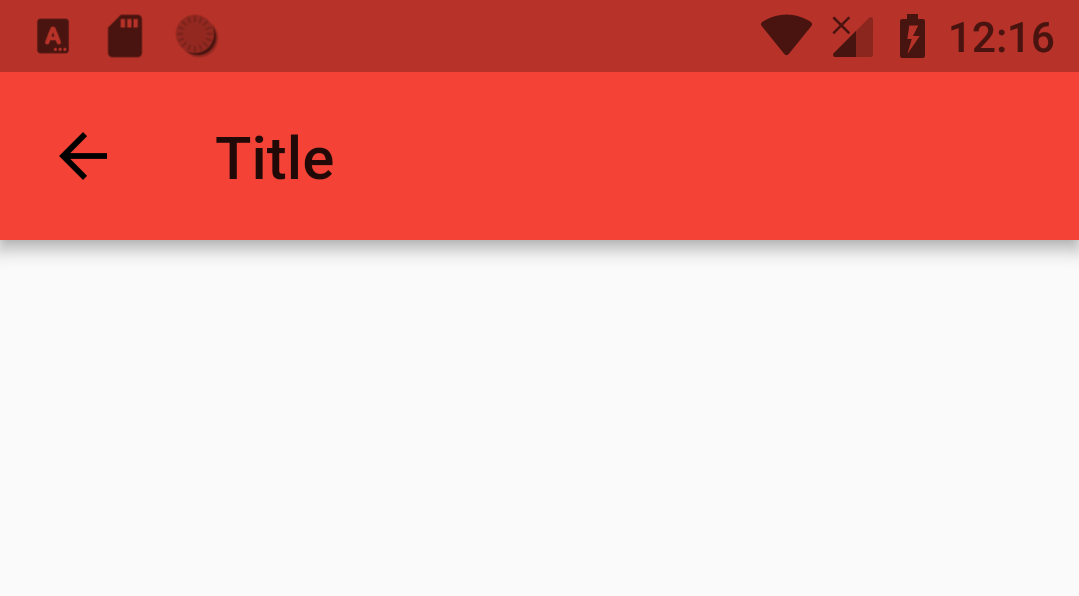 |
AppBar with List of Actions |
AppBar(
title: new Text("Title"),
actions: ≶Widget>[
new IconButton(
icon: new Icon(Icons.search),
onPressed: () {},
),
new IconButton(
icon: new Icon(Icons.add),
onPressed: () {},
),
],
),
| 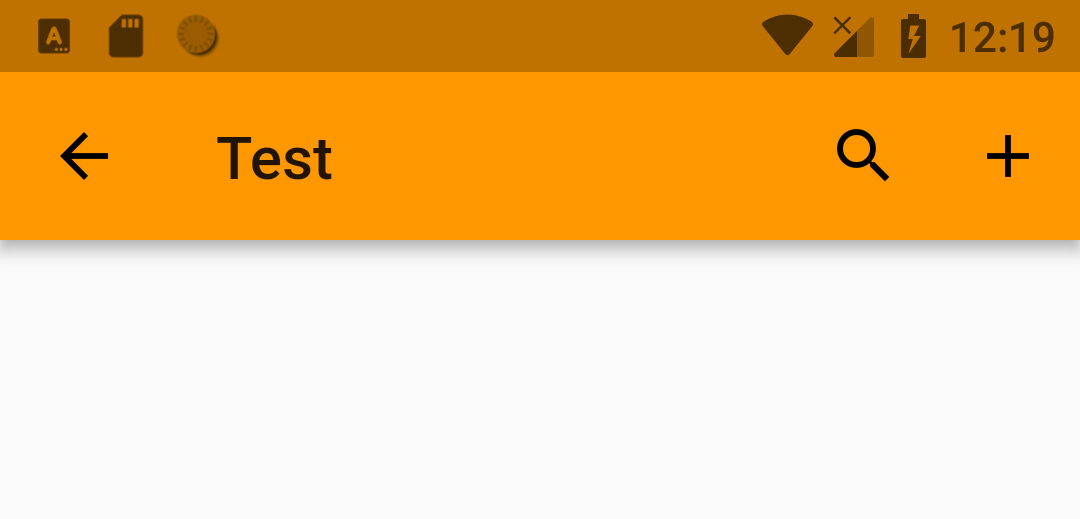 |
AppBar with Text and Icon Themes |
AppBar(
backgroundColor: Colors.blueAccent,
title: new Text("Title"),
actions: ≶Widget>[
new IconButton(
icon: new Icon(Icons.search),
onPressed: () {},
),
],
iconTheme: IconThemeData(
color: Colors.white,
),
textTheme: TextTheme(
title: TextStyle(
color: Colors.white,
fontSize: 20.0
),
),
),
| 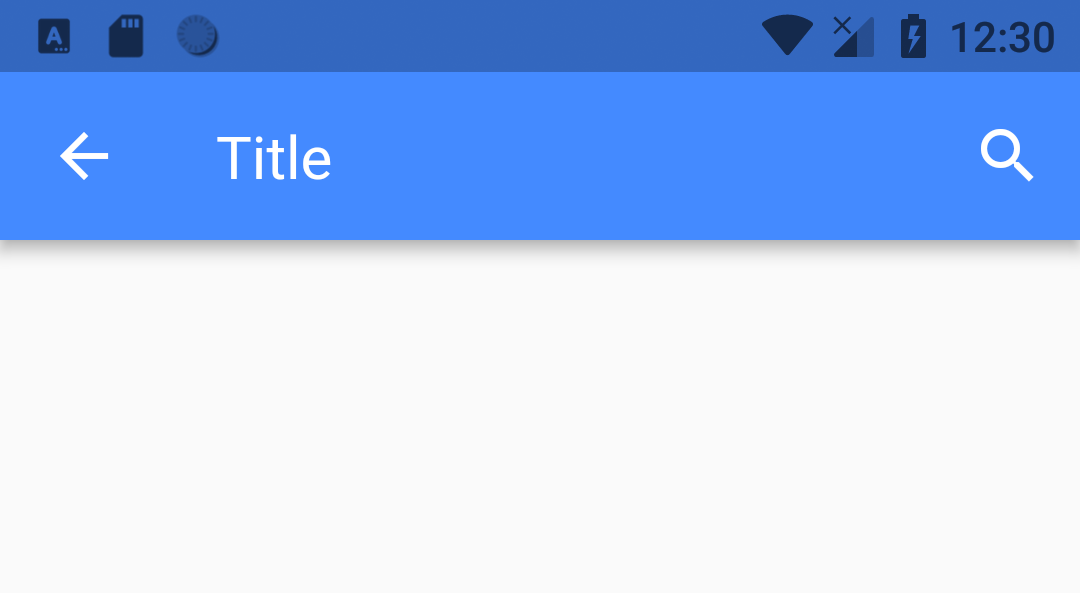 |
AppBar with centered Title and Subtitle |
AppBar(
automaticallyImplyLeading: false,
title: Center(
child: Column(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisSize: MainAxisSize.max,
mainAxisAlignment: MainAxisAlignment.center,
children: ≶Widget>[
new Text(
"Title",
style: TextStyle(fontSize: 18.0),
),
new Text(
"subtitle",
style: TextStyle(fontSize: 14.0),
),
],
),
),
),
| 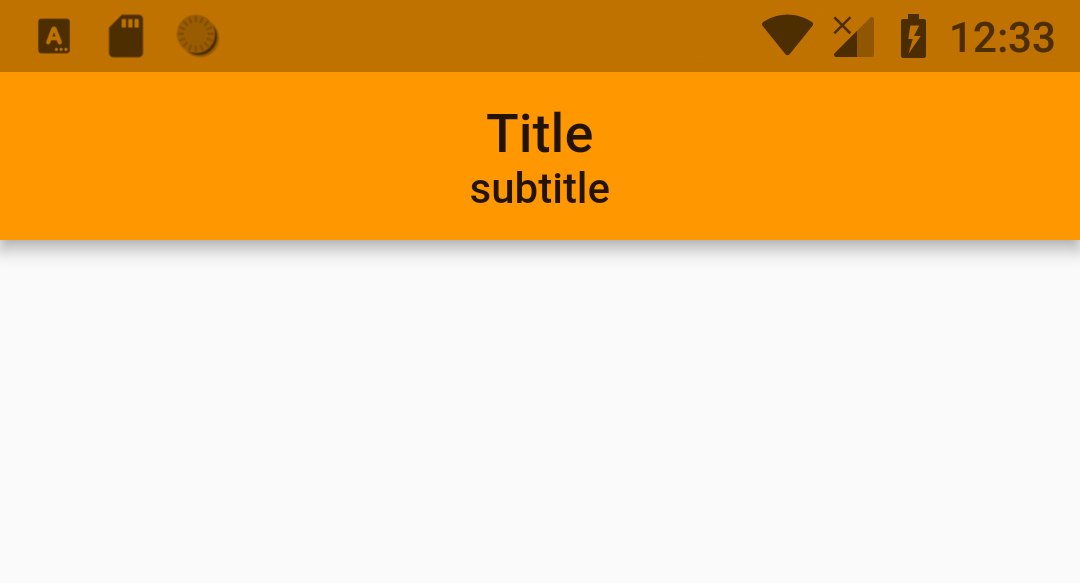 |
AppBar with Logo |
AppBar(
automaticallyImplyLeading: false,
backgroundColor: Colors.yellow,
title: Row(
mainAxisAlignment: MainAxisAlignment.start,
mainAxisSize: MainAxisSize.max,
children: ≶Widget>[
new FlutterLogo(),
Padding(
padding: const EdgeInsets.only(left: 16.0),
child: new Text(
"Title with image",
),
),
],
),
),
| 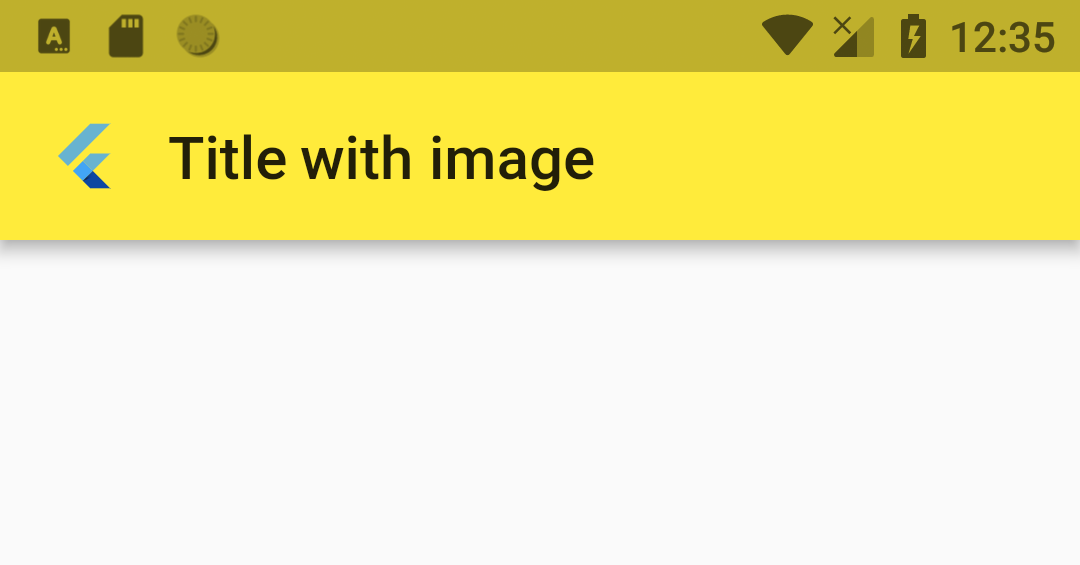 |
Transparent AppBar |
AppBar(
backgroundColor: Colors.transparent,
title: Text("Transparent AppBar"),
actions: ≶Widget>[
IconButton(
icon: Icon(
Icons.search,
),
onPressed: () {},
)
],
),
| 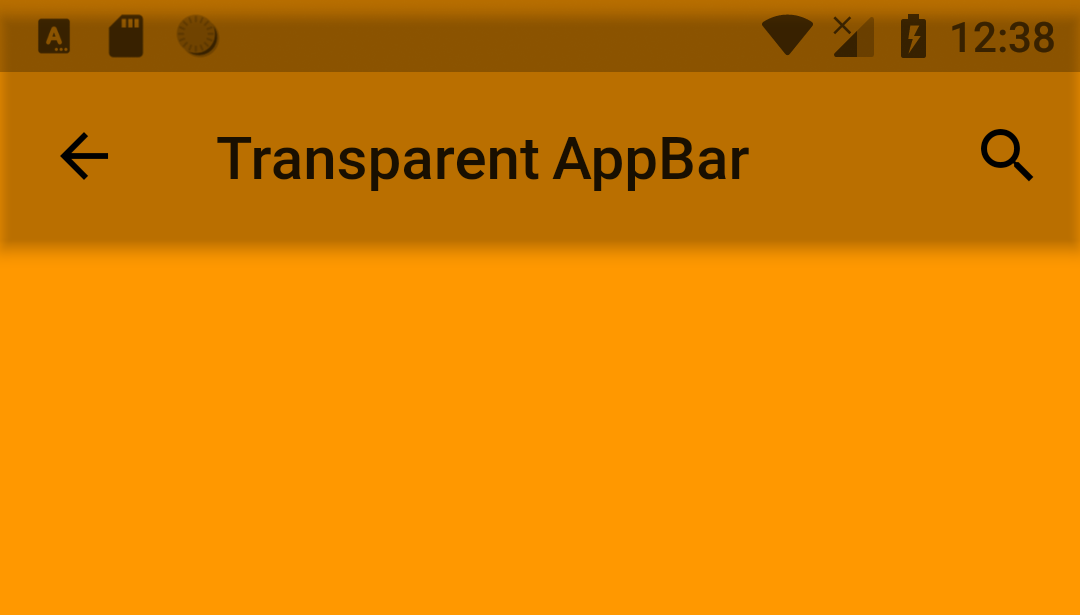 |
Container with full-device sized Flutter Logo |
Container(
height: MediaQuery.of(context).size.height,
width: MediaQuery.of(context).size.width,
margin: EdgeInsets.all(25.0),
decoration: FlutterLogoDecoration(),
),
| 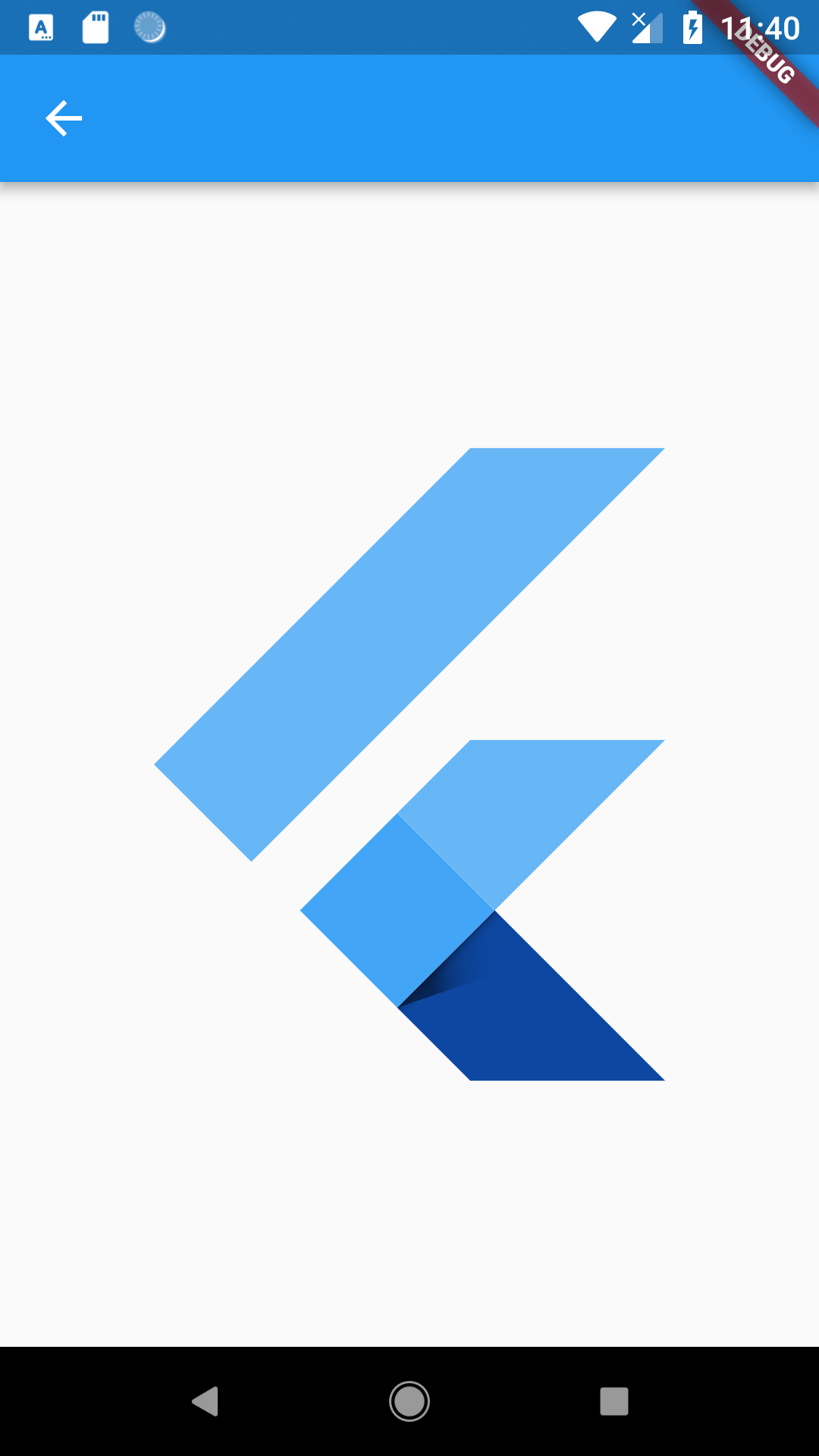 |
Container with shadow, border, and child |
Container(
height: MediaQuery.of(context).size.height,
width: MediaQuery.of(context).size.width,
margin: EdgeInsets.all(25.0),
decoration: new ShapeDecoration(
color: Colors.white,
shadows: ≶BoxShadow>[
BoxShadow(color: Colors.black, blurRadius: 15.0)
],
shape: new Border.all(
color: Colors.red,
width: 8.0,
),
),
child: Center(child: const Text('Hello Flutter', textAlign: TextAlign.center)),
),
| 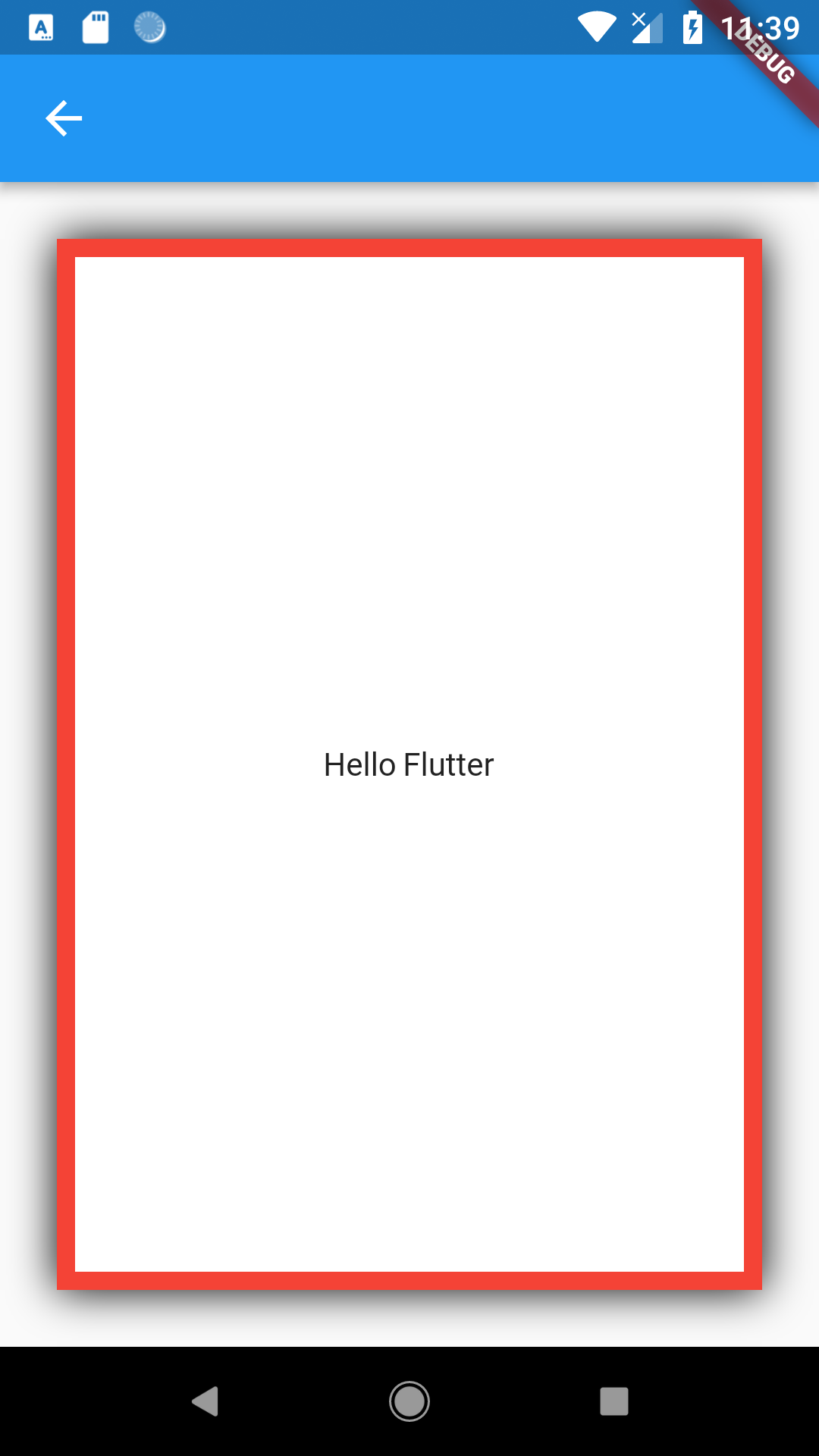 |
Rounded rectangle containers with border |
Container(
height: MediaQuery.of(context).size.height,
width: MediaQuery.of(context).size.width,
margin: EdgeInsets.all(25.0),
decoration: new BoxDecoration(
color: Colors.yellow,
borderRadius: new BorderRadius.circular(55.0),
border: new Border.all(
width: 5.0,
color: Colors.red,
),
),
child: Center(child: const Text('Hello Flutter', textAlign: TextAlign.center)),
),
| 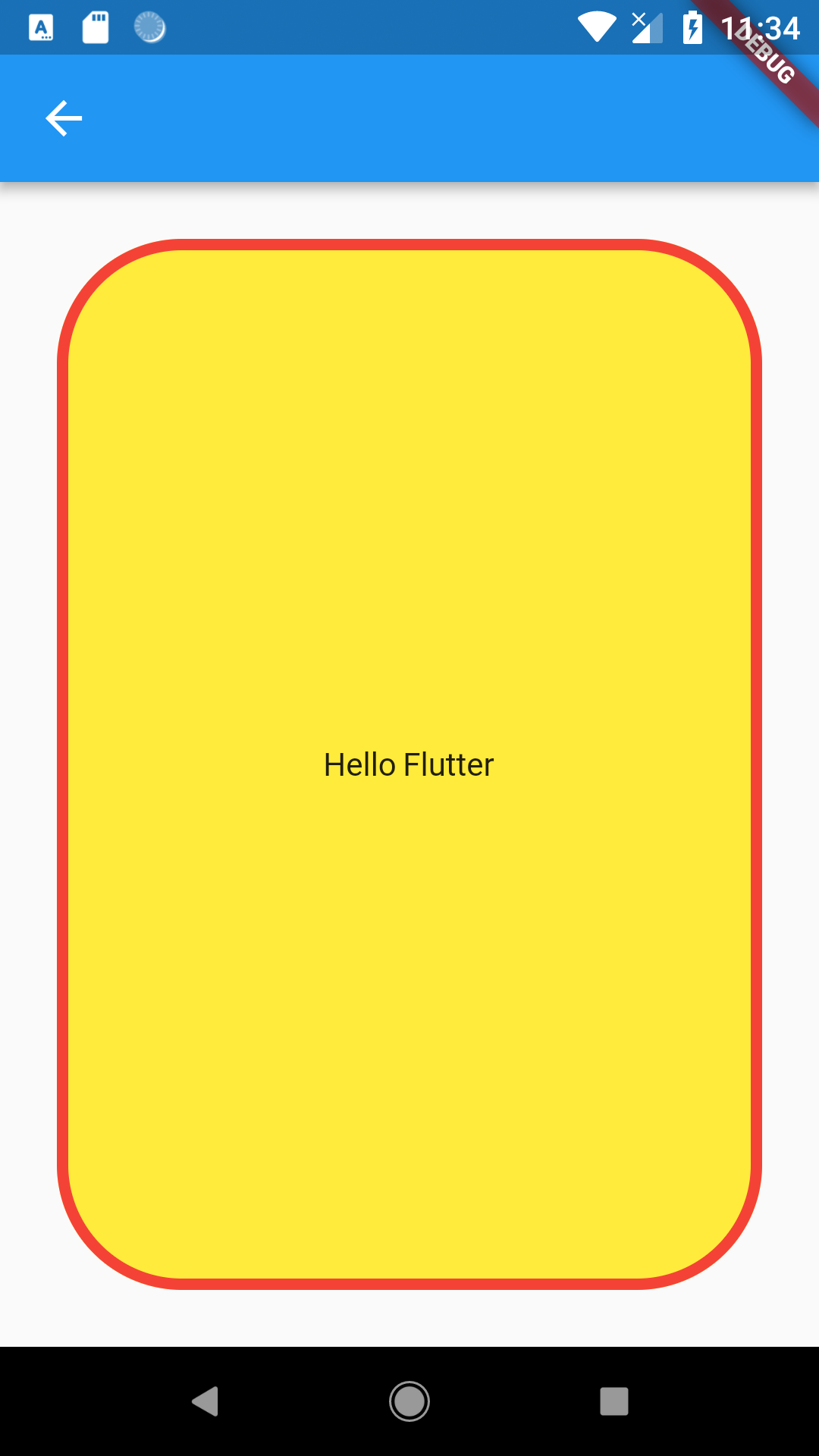 |
Simple Column of similar Text children |
Column(
children: ≶Widget>[
Text("Column 1", style: bigStyle,),
Text("Column 2", style: bigStyle,),
Text("Column 3", style: bigStyle,)
],
)
| 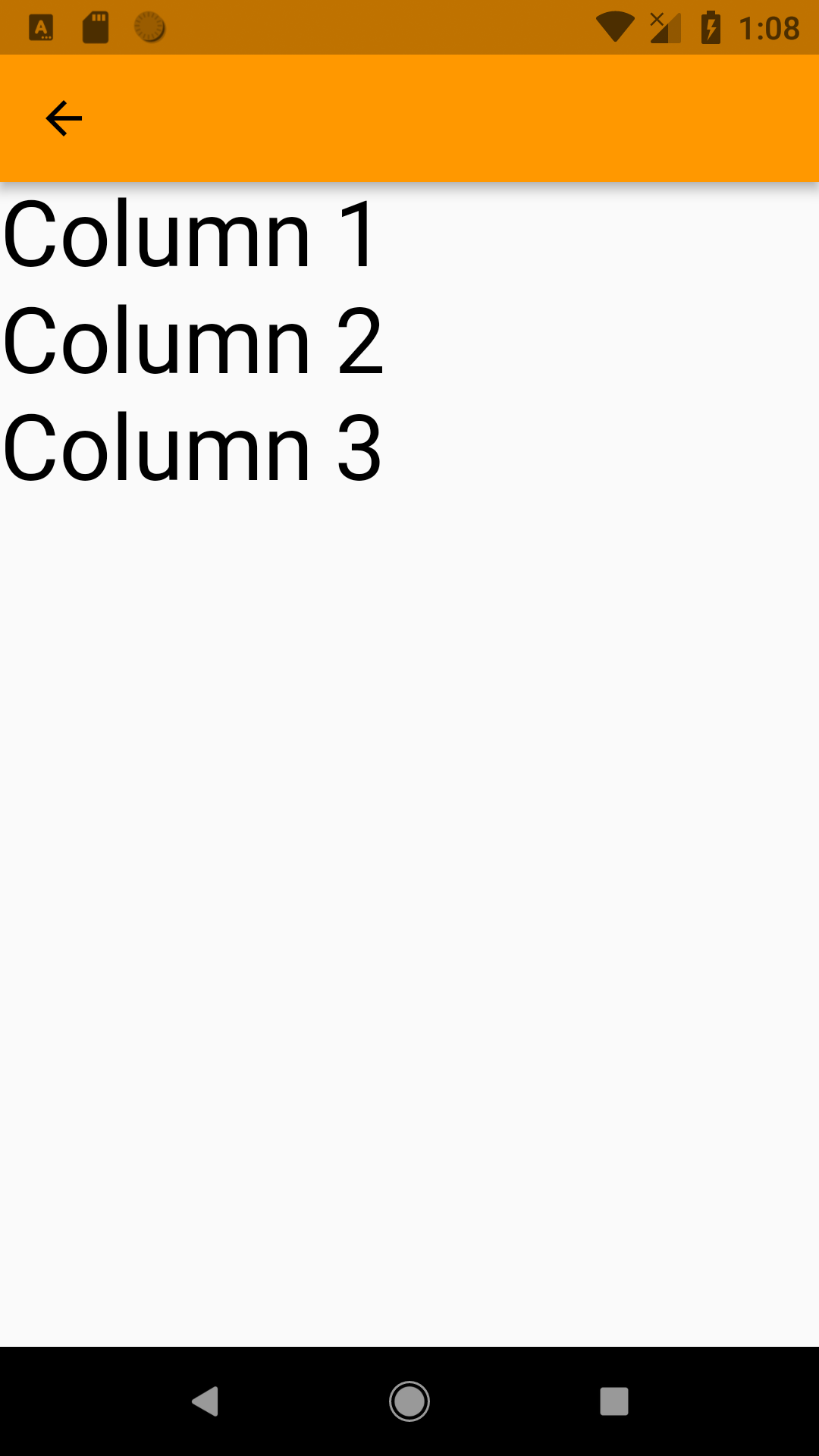 |
Column of different Widget children |
Column(
children: ≶Widget>[
FlutterLogo(
size: 100.0,
colors: Colors.red,
),
Text("Column 2", style: bigStyle,),
Container(
color: Colors.green,
height: 100.0,
width: 100.0,
)
],
)
| 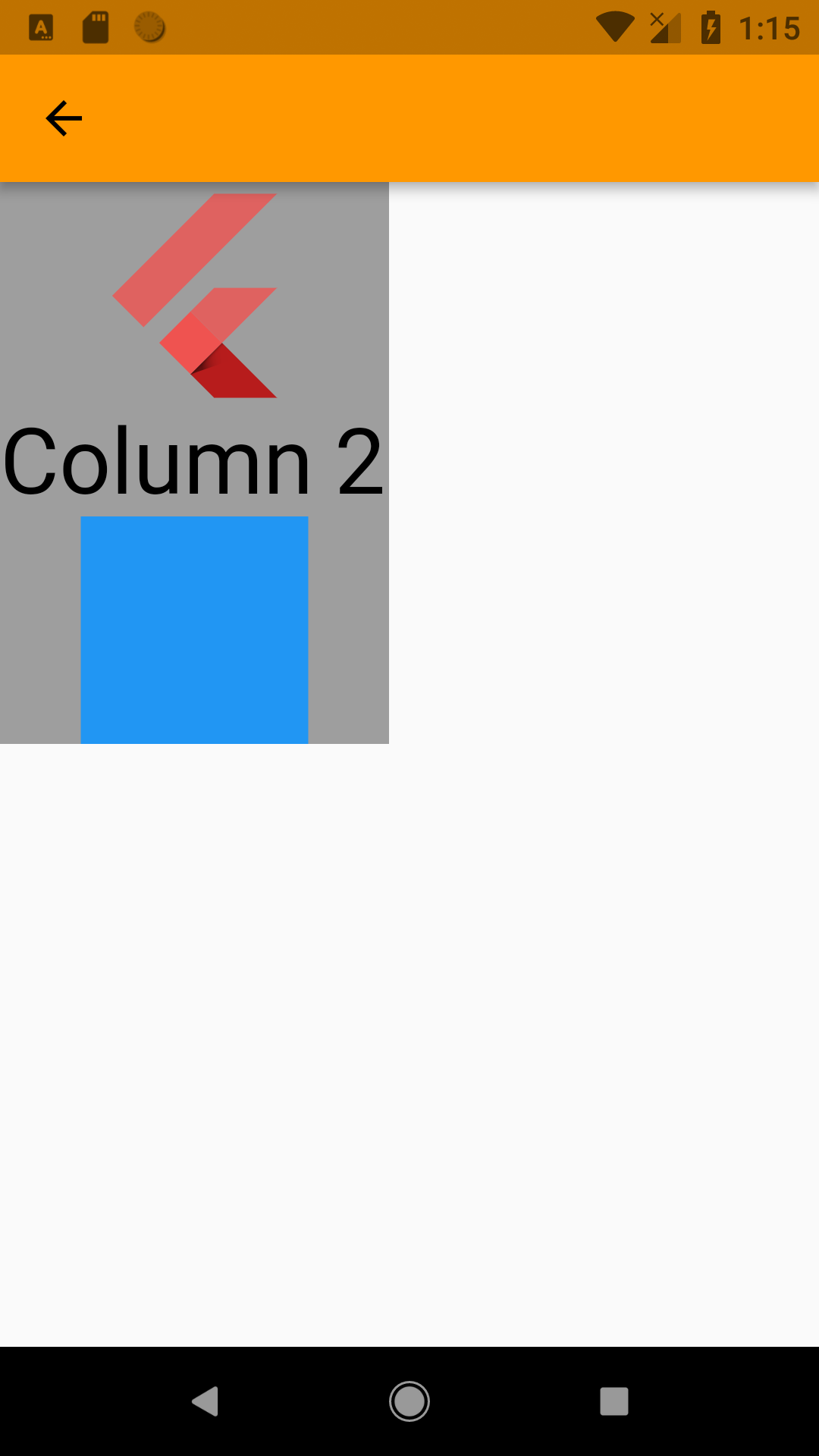 |
Playing with MainAxisAlignment |
Column(
mainAxisSize: MainAxisSize.max,
mainAxisAlignment: MainAxisAlignment.spaceAround,
crossAxisAlignment: CrossAxisAlignment.end,
children: ≶Widget>[
FlutterLogo(
size: 100.0,
colors: Colors.red,
),
Text("Child Two", style: bigStyle,),
Container(
color: Colors.blue,
height: 100.0,
width: 100.0,
)
],
),
| 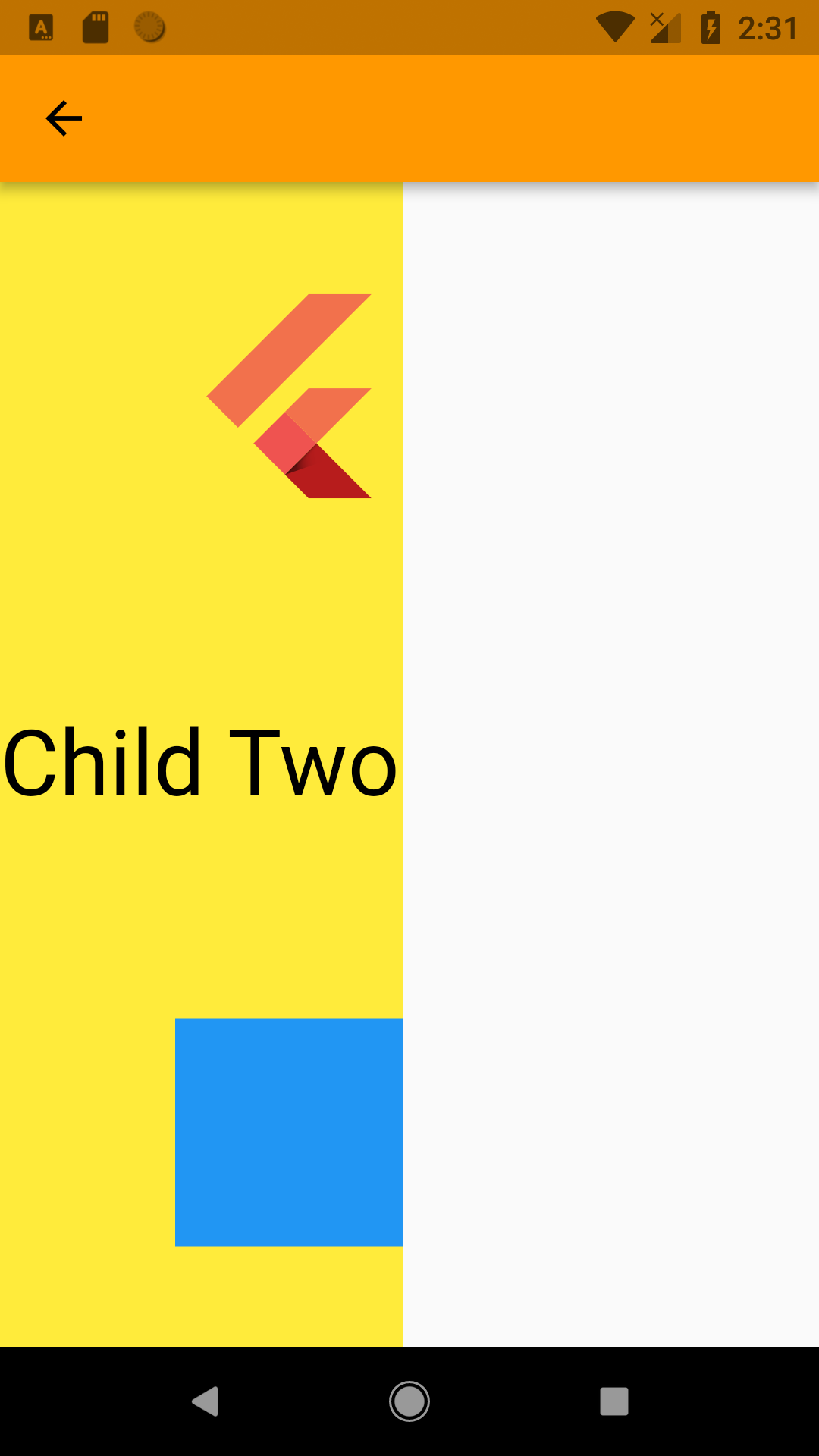 |
Column having Row as child |
Column(
mainAxisSize: MainAxisSize.max,
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: ≶Widget>[
Text("Parent Text 1"),
Text("Parent Text 2"),
Row(
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: ≶Widget>[
Text("Child Row Text 1"),
Text("Child Row Text 2")
],
),
],
),
| 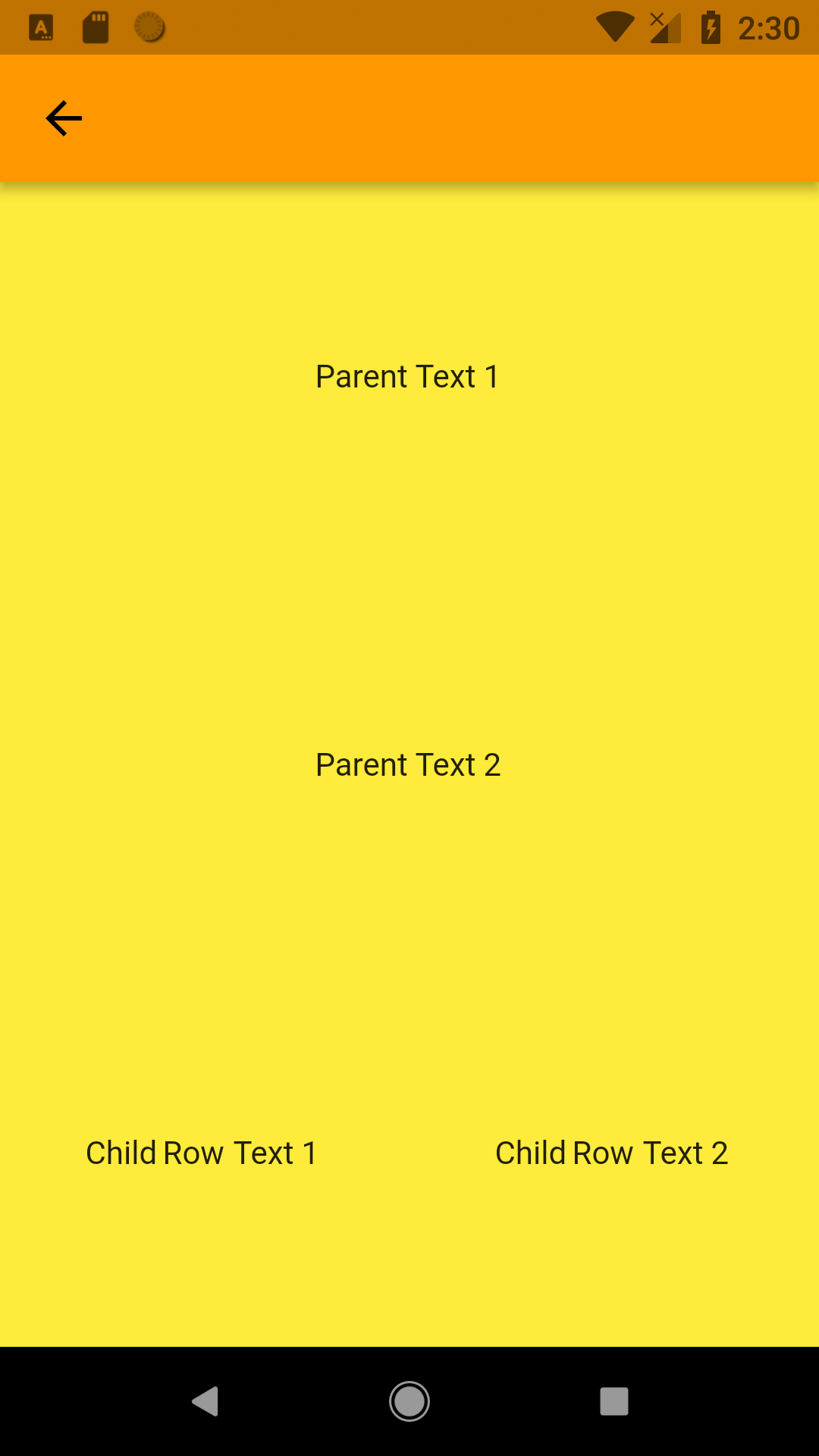 |
Simple Row of similar Text children |
Row(
mainAxisSize: MainAxisSize.min,
children: ≶Widget>[
Text("Column 1", style: bigStyle,),
Text("Column 2", style: bigStyle,),
Text("Column 3", style: bigStyle,)
],
)
| 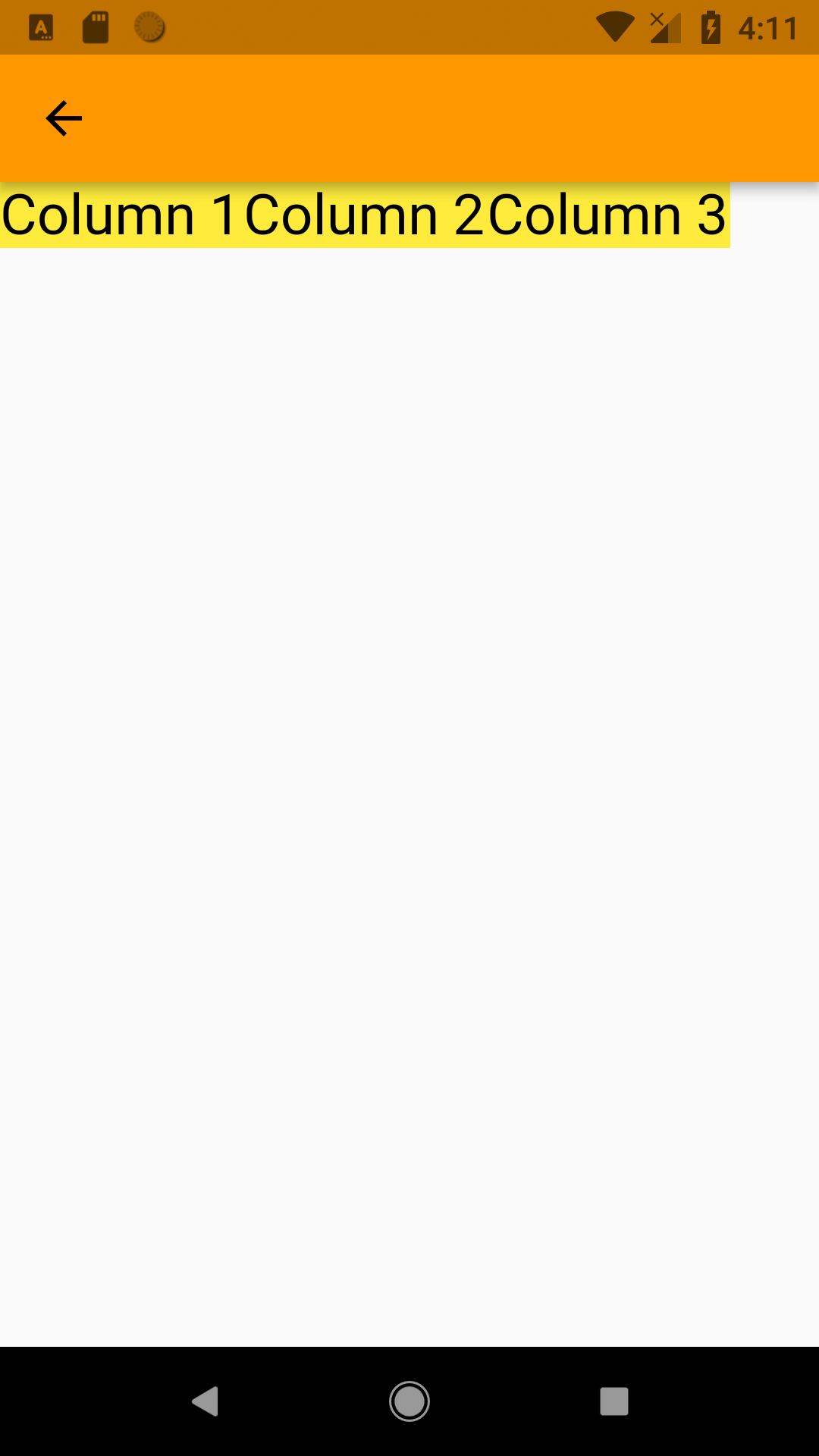 |
Row with children of different Widgets |
Row(
mainAxisSize: MainAxisSize.min,
children: ≶Widget>[
FlutterLogo(
size: 100.0,
colors: Colors.red,
),
Text("Column 2", style: bigStyle,),
Container(
color: Colors.green,
height: 100.0,
width: 100.0,
)
],
)
| 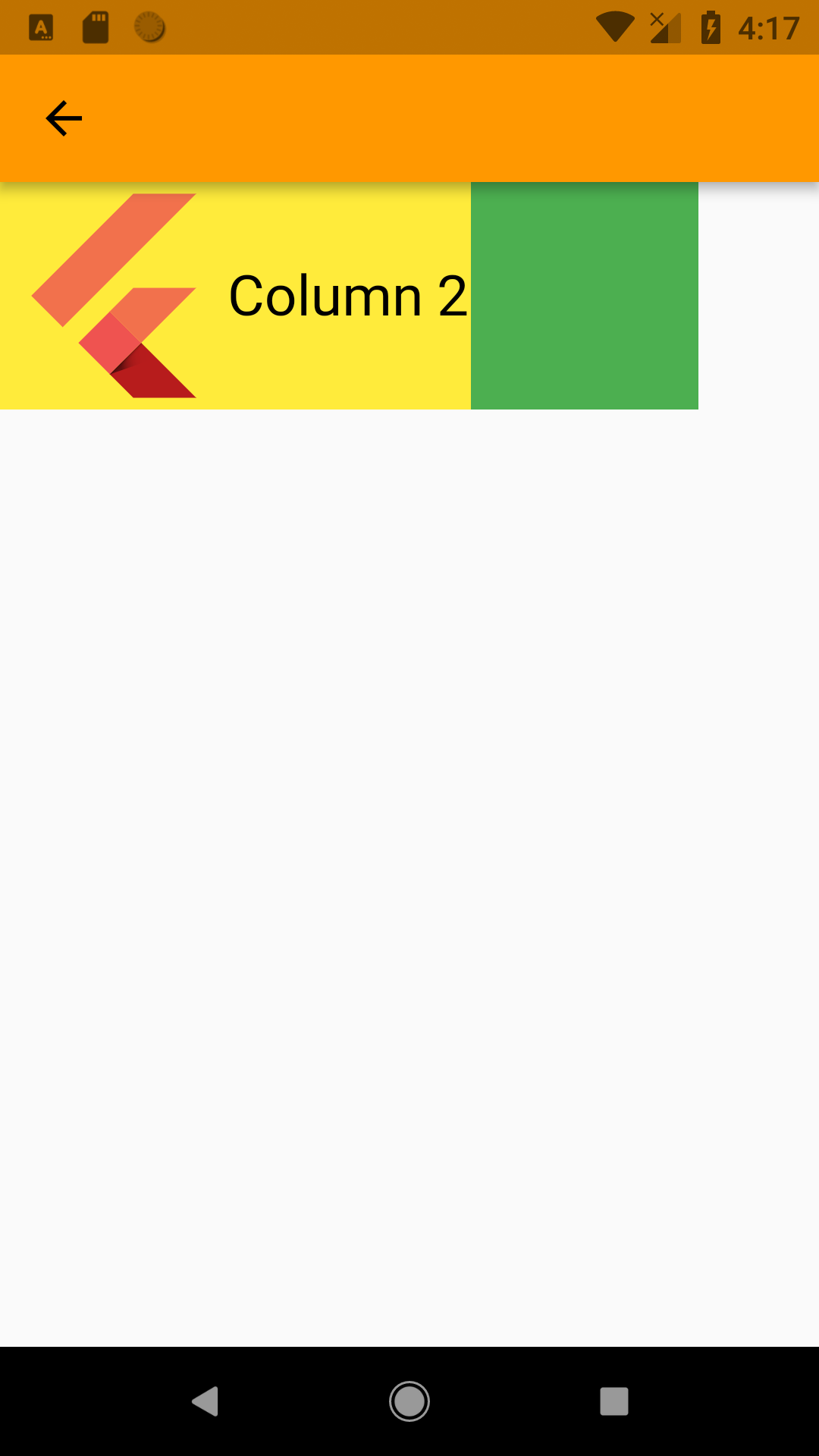 |
Playing with MainAxisAlignment |
Row(
mainAxisSize: MainAxisSize.max,
mainAxisAlignment: MainAxisAlignment.spaceBetween,
crossAxisAlignment: CrossAxisAlignment.center,
children: ≶Widget>[
FlutterLogo(
size: 100.0,
colors: Colors.red,
),
Text("Child Two", style: bigStyle,),
Container(
color: Colors.blue,
height: 100.0,
width: 100.0,
)
],
),
| 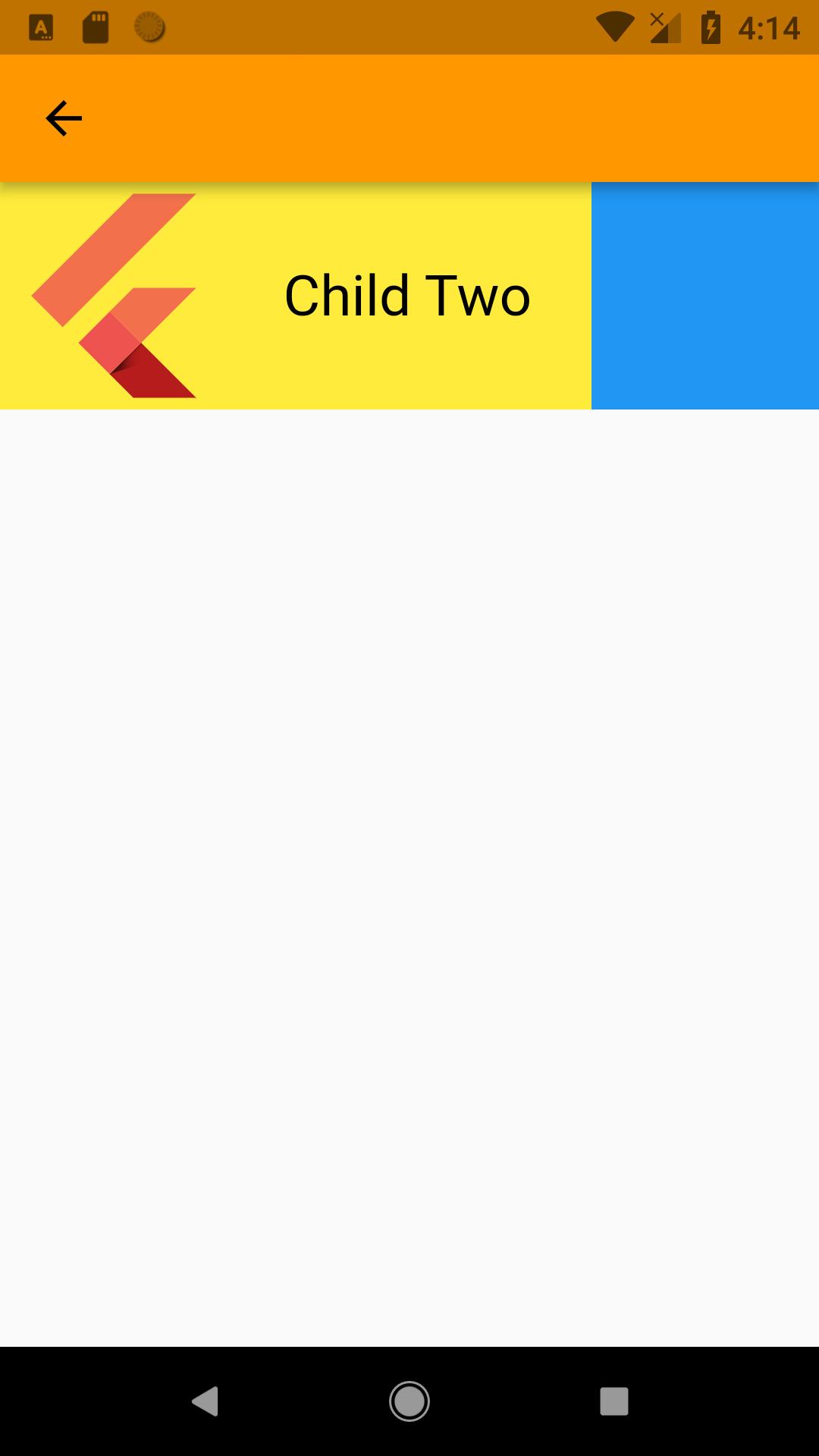 |
Row having Column as child |
Row(
mainAxisSize: MainAxisSize.min,
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: ≶Widget>[
Text("Parent Text 1"),
Text("Parent Text 2"),
Column(
mainAxisSize: MainAxisSize.min,
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: ≶Widget>[
Text("Child Row Text 1"),
Text("Child Row Text 2")
],
),
],
),
| 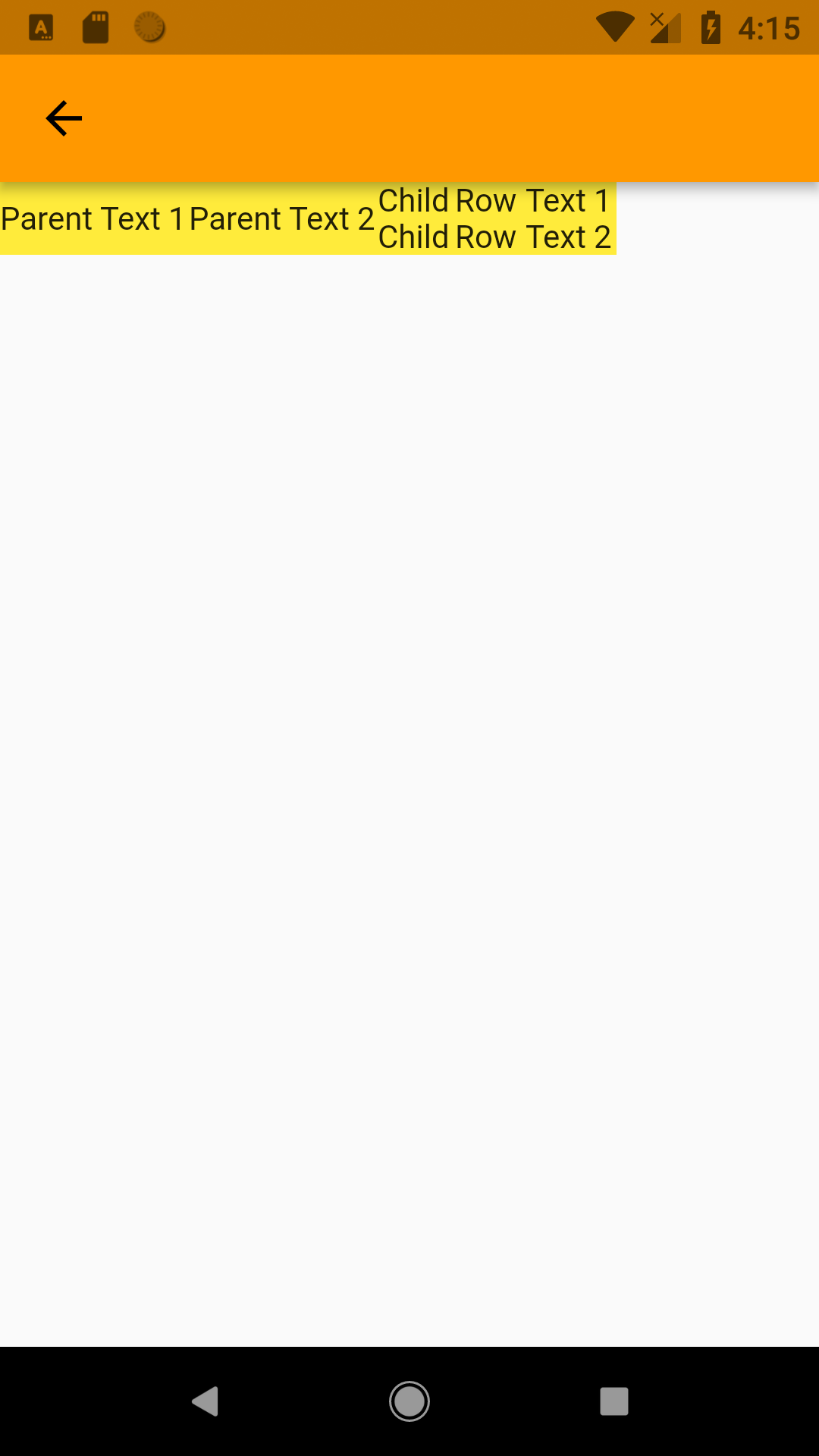 |
RaisedButton(
onPressed: (){},
color: Colors.yellow,
disabledTextColor: Colors.grey,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(20.0)
),
elevation: 20.0,
splashColor: Colors.green,
highlightColor: Colors.red,
highlightElevation: 1.0,
child: Text("Raised Button"),
),
| 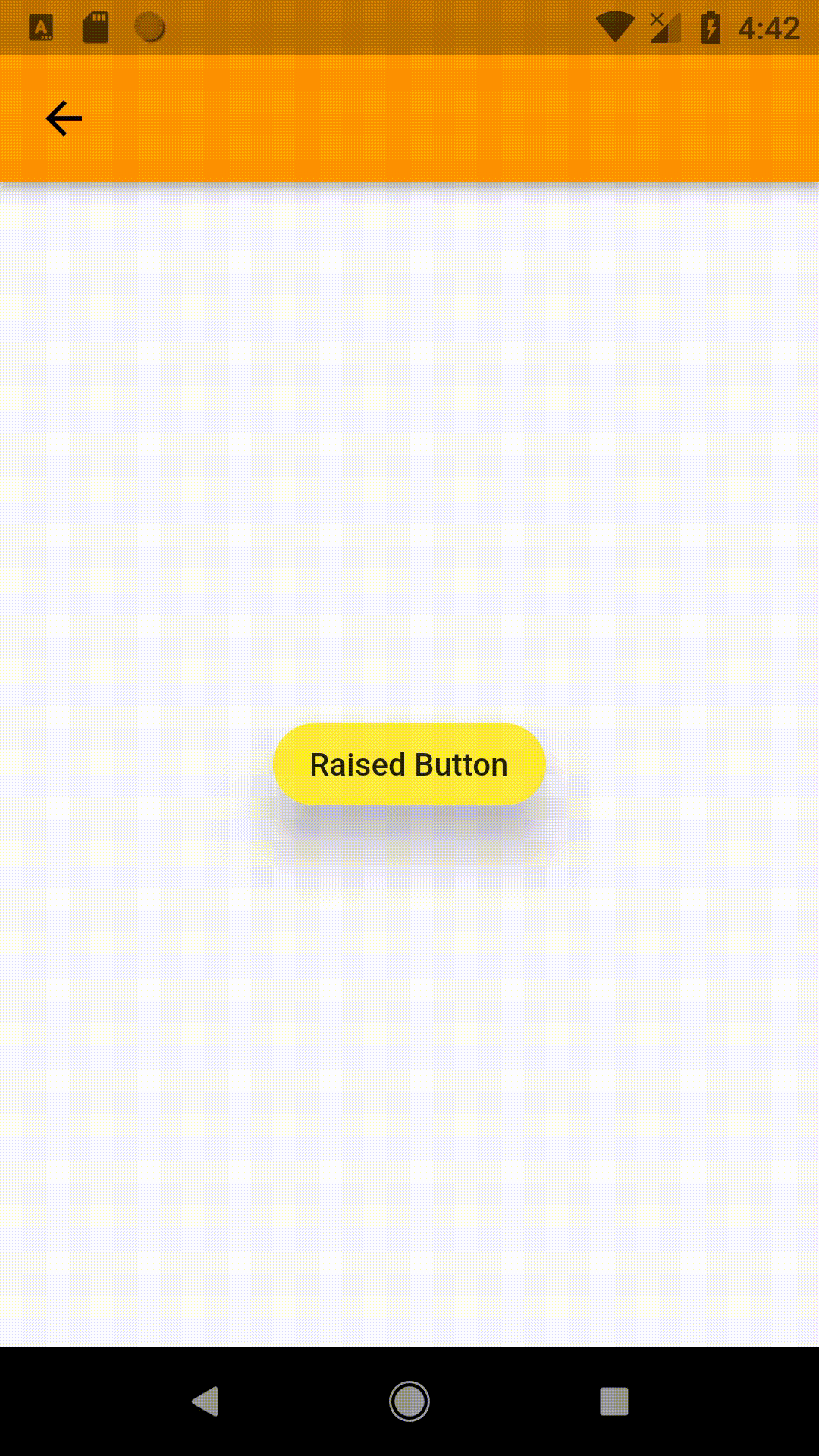 |
MaterialButton(
minWidth: 250.0,
onPressed: (){},
colorBrightness: Brightness.dark,
color: Colors.deepPurpleAccent,
elevation: 20.0,
splashColor: Colors.green,
//highlightColor: Colors.red,
highlightElevation: 1.0,
child: Text("Material Button"),
),
| 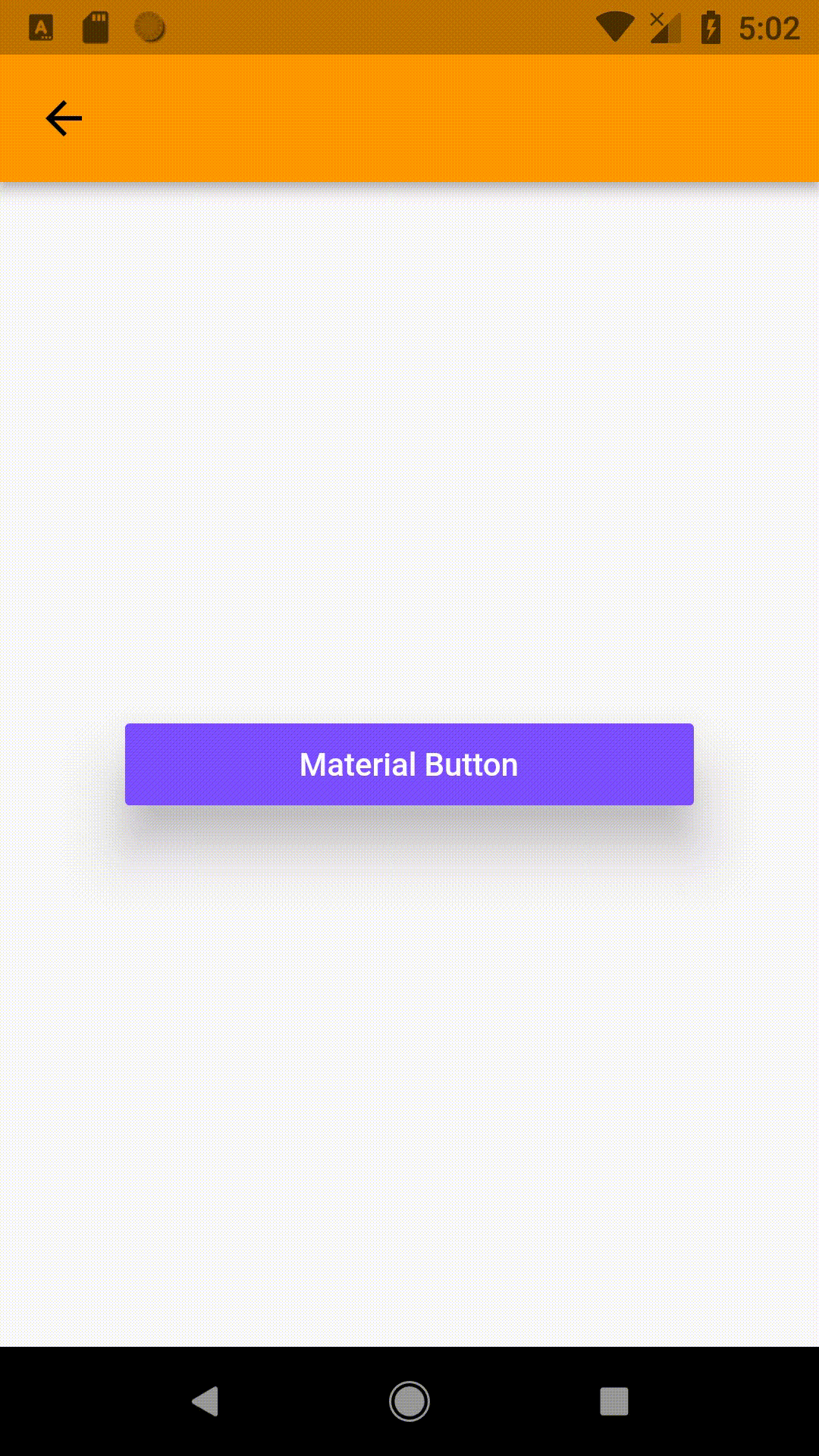 |
FlatButton(
onPressed: (){},
colorBrightness: Brightness.dark,
color: Colors.deepPurpleAccent,
splashColor: Colors.green,
highlightColor: Colors.red,
child: Text("Raised Button"),
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.horizontal(
left: Radius.circular(20.0), right: Radius.circular(1.0))
),
),
| 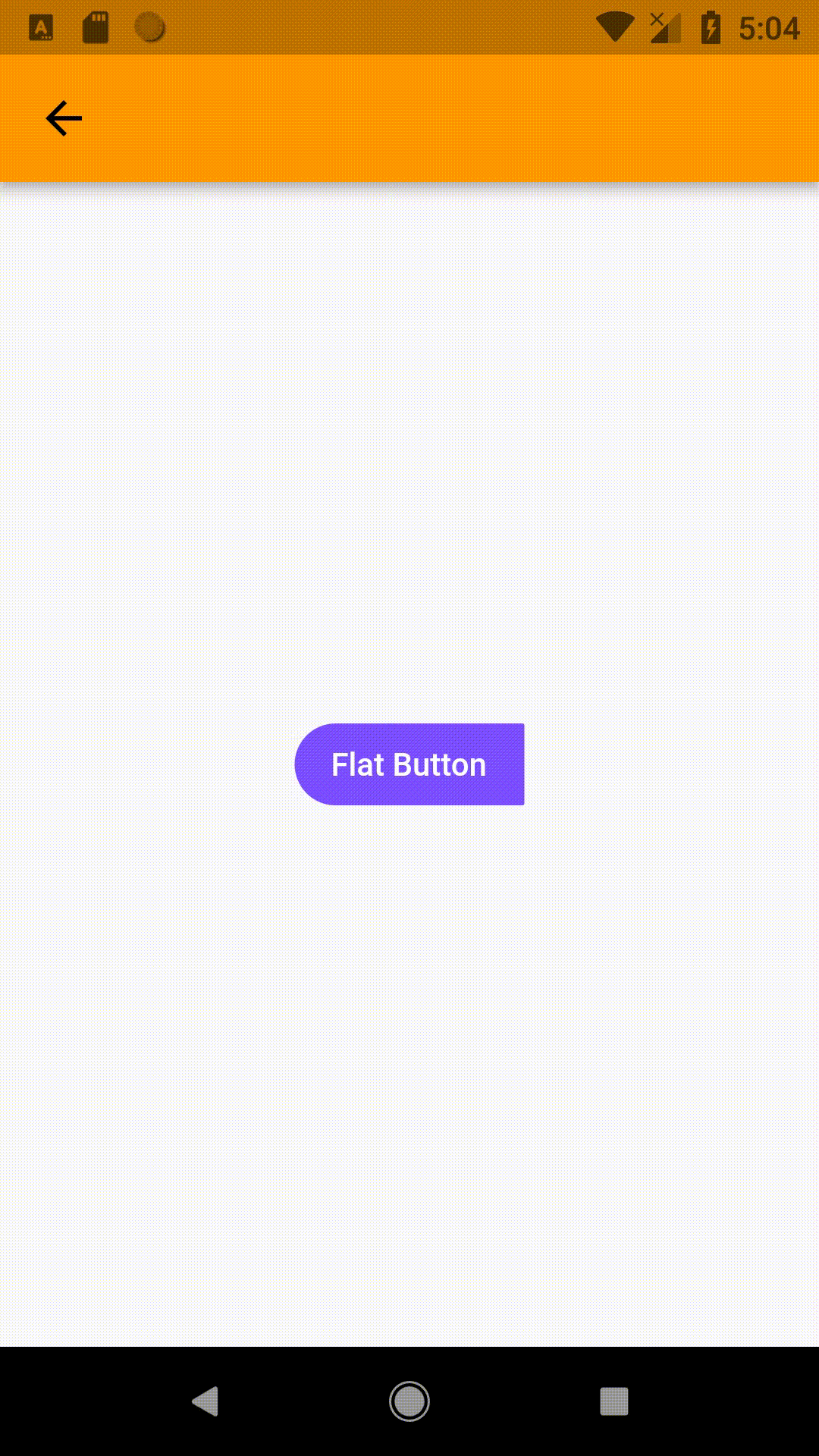 |
OutlineButton(
onPressed: (){},
borderSide: BorderSide(
width: 5.0,
color: Colors.deepPurpleAccent
),
color: Colors.deepPurpleAccent,
highlightedBorderColor: Colors.purple,
splashColor: Colors.green,
//highlightColor: Colors.red,
child: Text("Raised Button"),
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.vertical(
top: Radius.circular(20.0), bottom: Radius.circular(1.0))
),
),
| 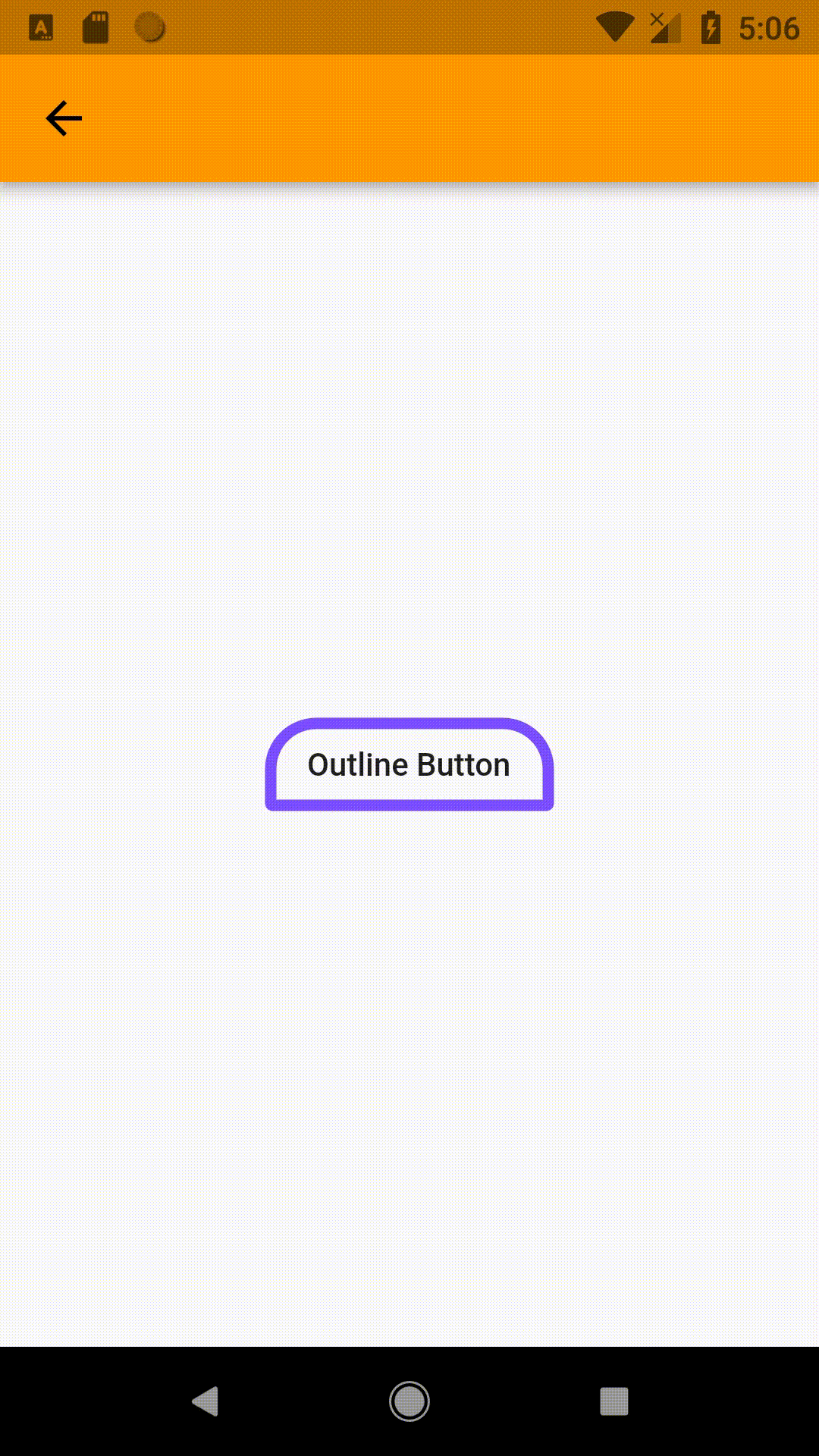 |
IconButton(
color: Colors.purple,
splashColor: Colors.yellow,
// highlightColor: Colors.red,
icon: Icon(Icons.build, size: 40.0,),
onPressed: (){})
),
| 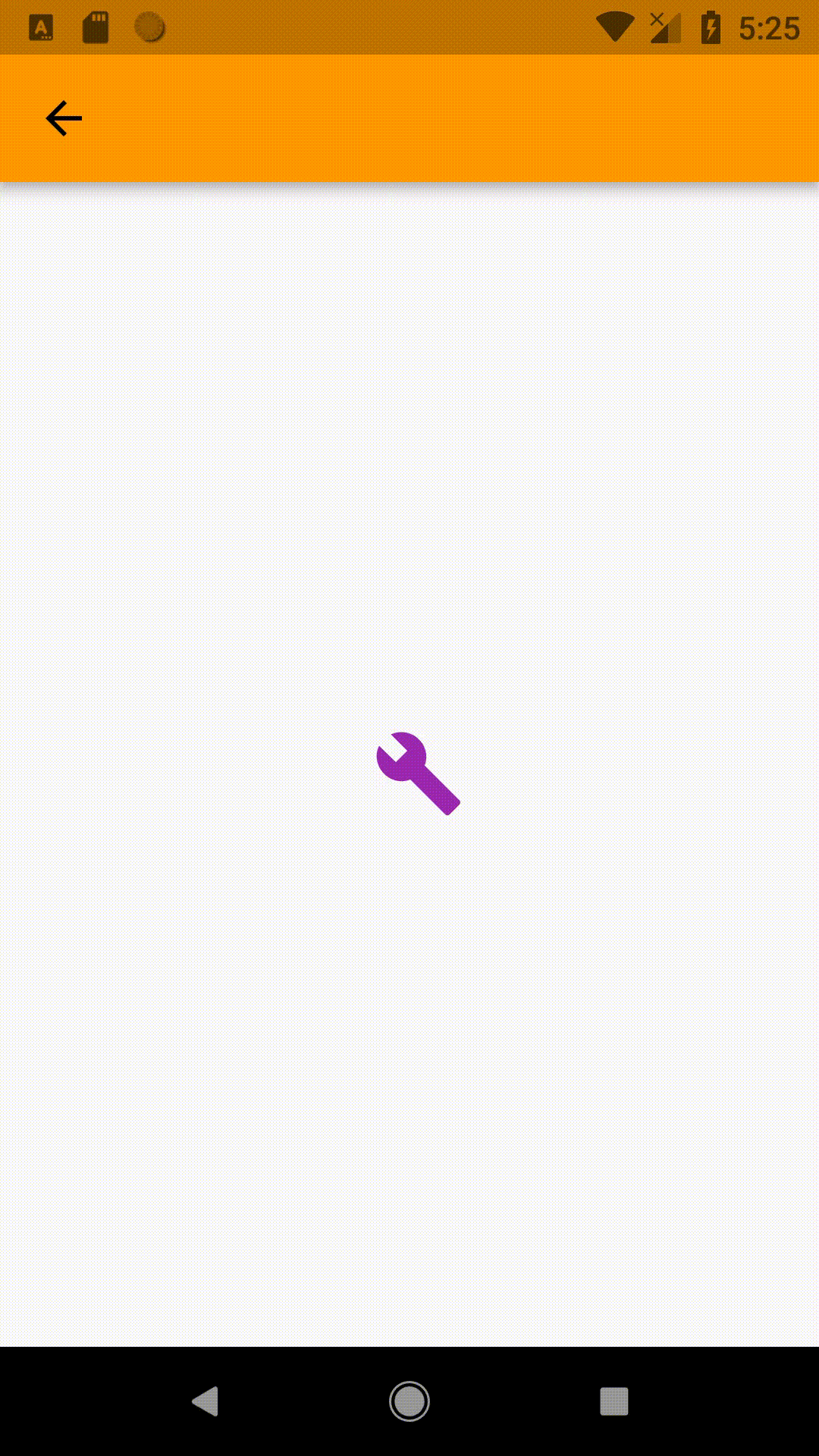 |
Stack of overlapping containers of reducing size |
Stack(
children: ≶Widget>[
Container(
height: 300.0,
width: 300.0,
color: Colors.red,
),
Container(
height: 250.0,
width: 250.0,
color: Colors.green,
),
Container(
height: 200.0,
width: 200.0,
color: Colors.yellow,
)
],
),
| 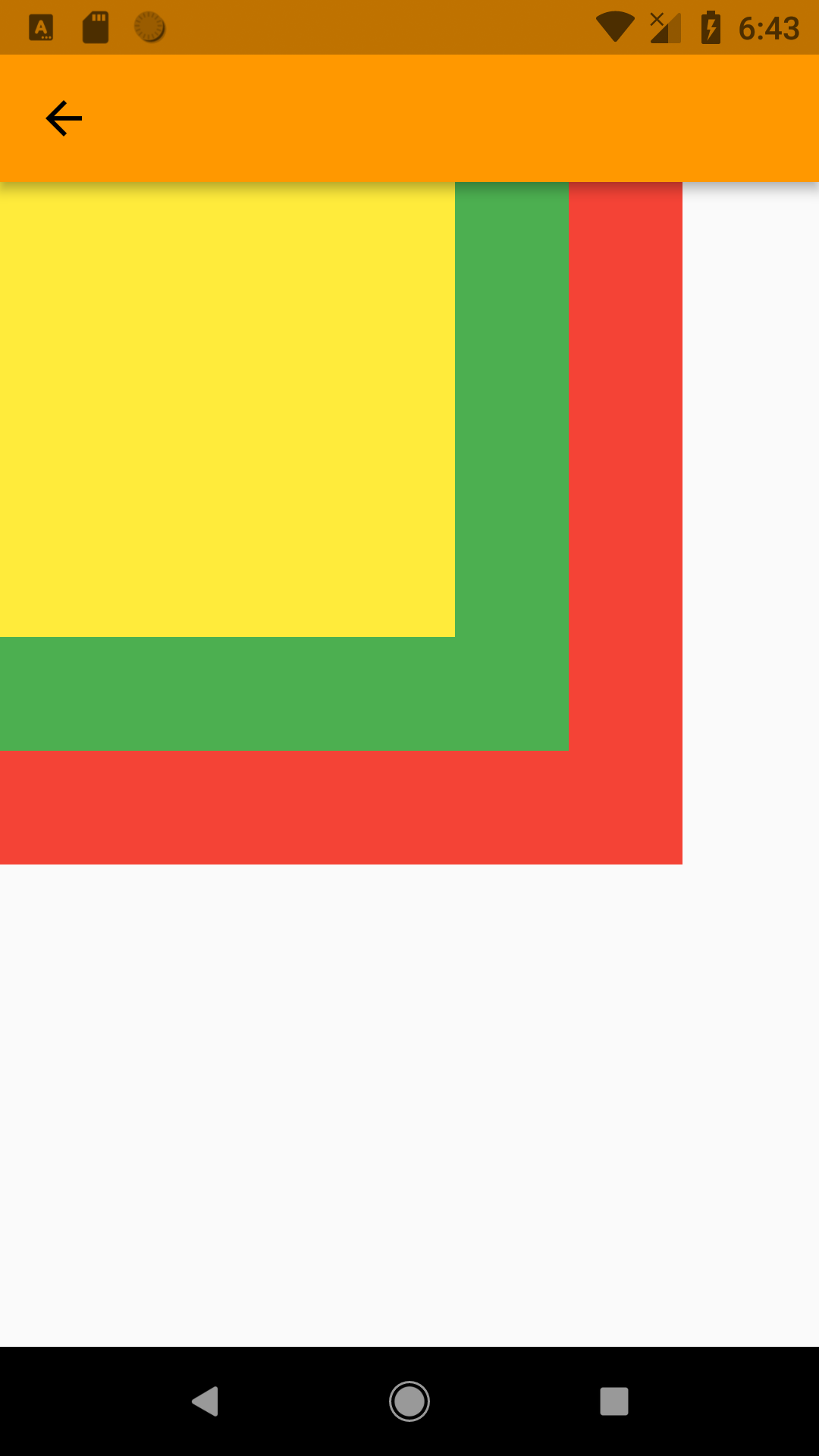 |
Playing with Alignment property |
Stack(
alignment: Alignment.center,
children: ≶Widget>[
Container(
height: 300.0,
width: 300.0,
color: Colors.red,
),
Container(
height: 250.0,
width: 250.0,
color: Colors.green,
),
Container(
height: 200.0,
width: 200.0,
color: Colors.yellow,
)
],
), | 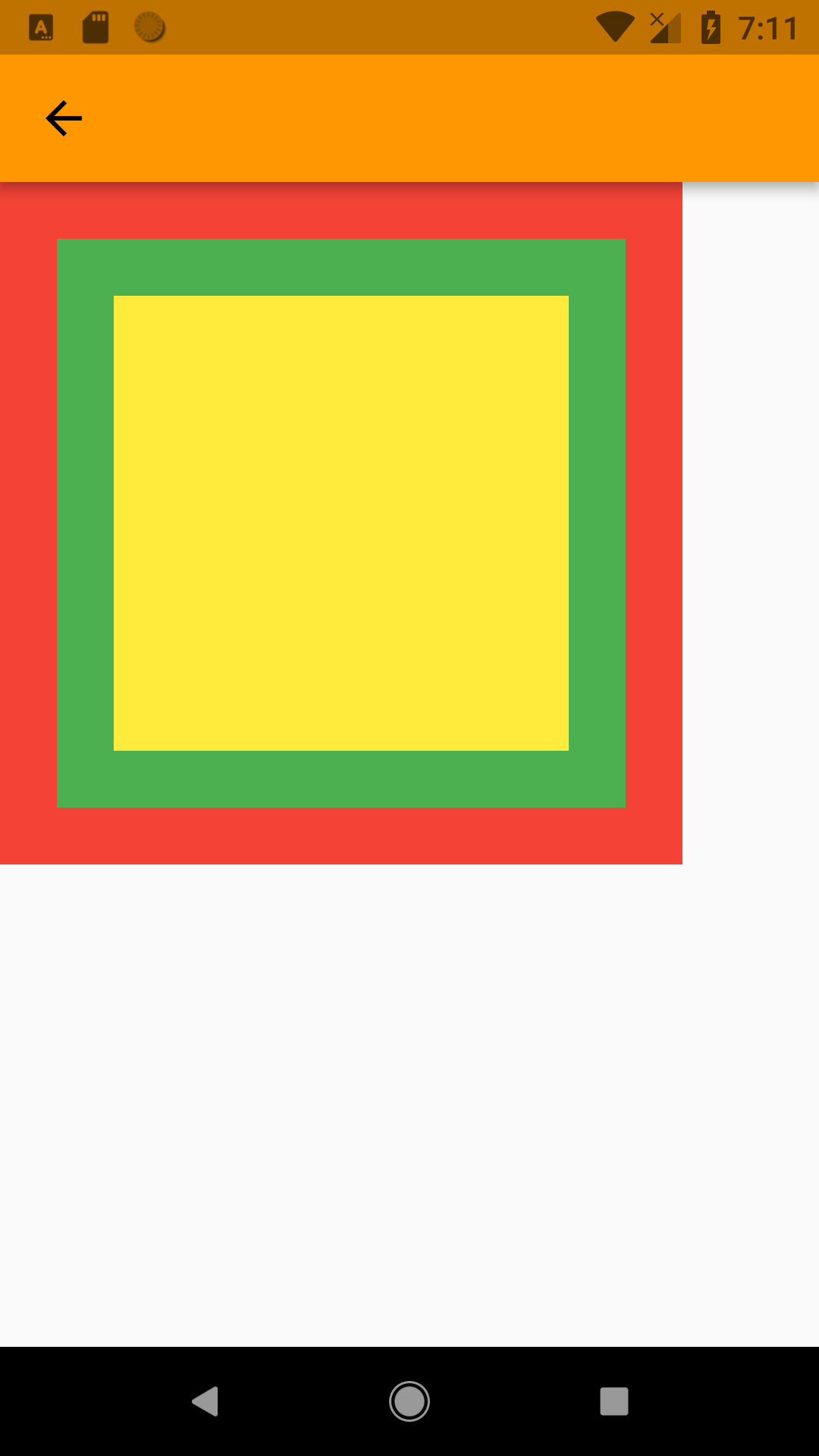 |
One child on top of another using Positioned |
Container(
height: 400.0,
//color: Colors.yellow,
child: Padding(
padding: const EdgeInsets.all(8.0),
child: Stack(
alignment: Alignment.center,
children: ≶Widget>[
Container(
height: 300.0,
width: 300.0,
color: Colors.red,
),
Positioned(
top: 0.0,
child: Container(
height: 250.0,
width: 250.0,
color: Colors.green,
),
),
],
),
),
),
| 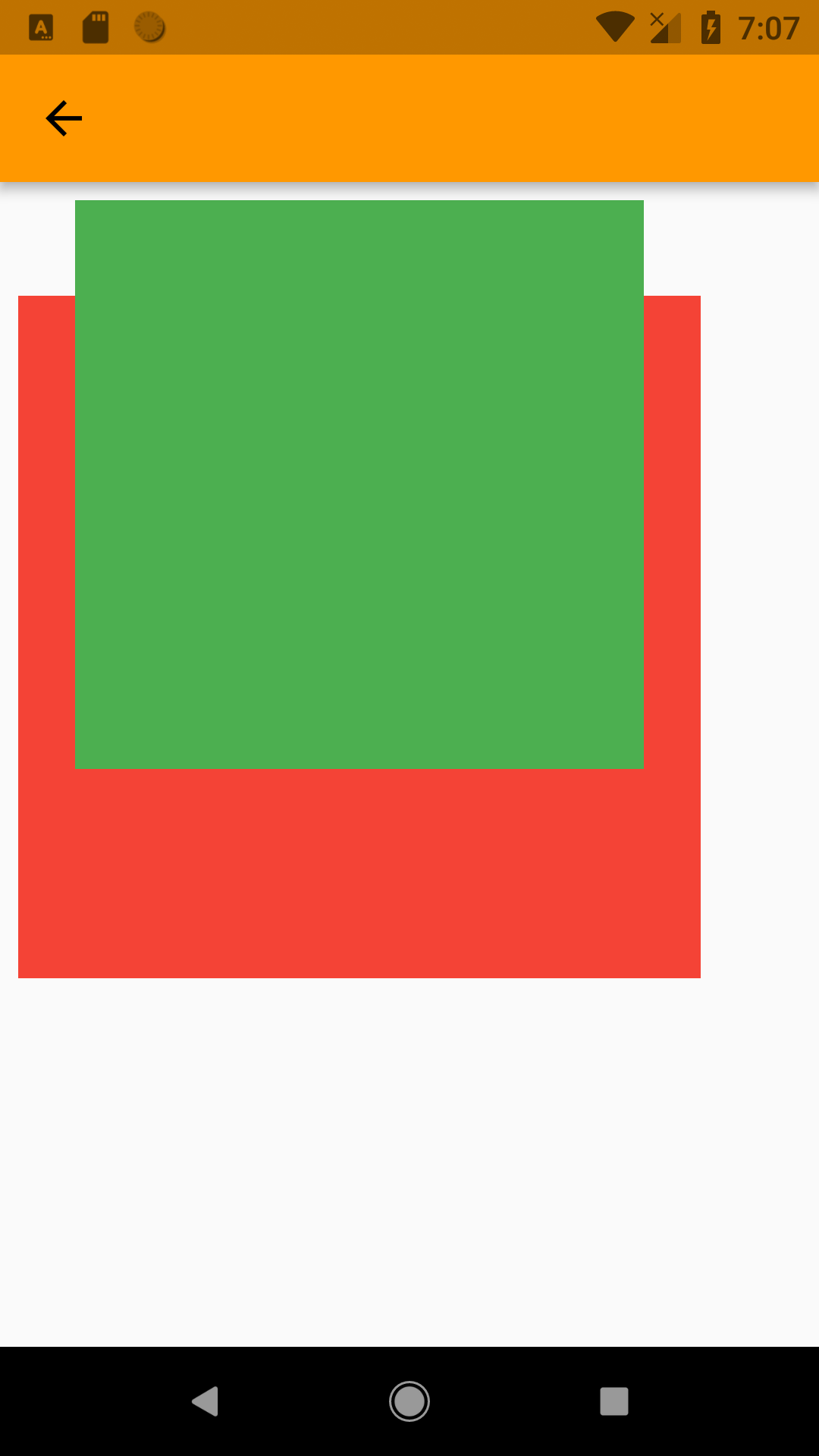 |
Playing with Positioned properties |
Container(
height: 400.0,
//color: Colors.yellow,
child: Padding(
padding: const EdgeInsets.all(8.0),
child: Stack(
alignment: Alignment.center,
children: ≶Widget>[
Container(
height: 300.0,
width: 300.0,
color: Colors.red,
),
Positioned(
top: 0.0,
bottom: 0.0,
child: Container(
height: 250.0,
width: 250.0,
color: Colors.green,
),
),
],
),
),
),
| 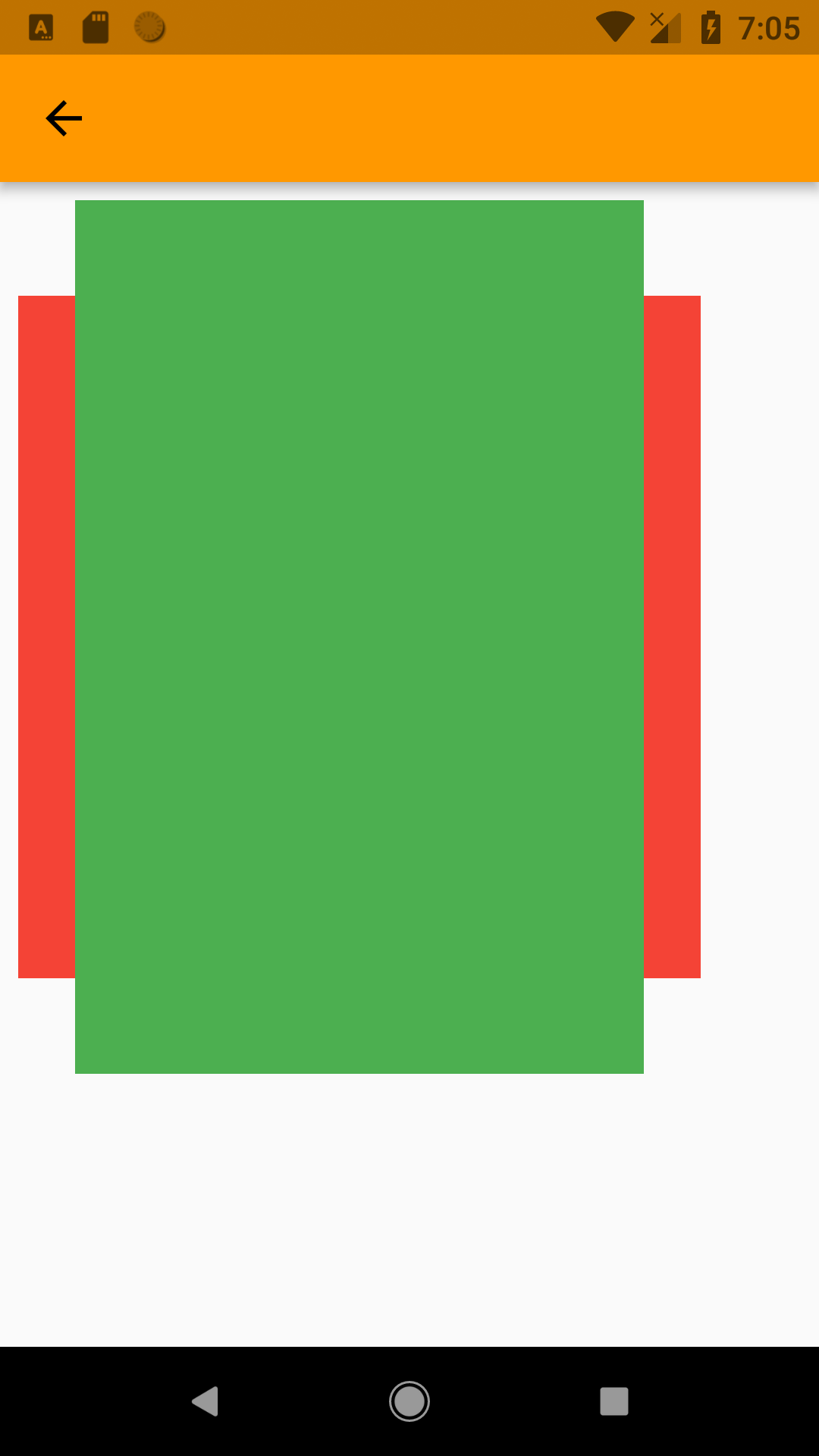 |
Playing with Positioned |
Container(
height: 400.0,
width: 350.0,
//color: Colors.yellow,
child: Padding(
padding: const EdgeInsets.all(8.0),
child: Stack(
alignment: Alignment.center,
children: ≶Widget>[
Container(
height: 300.0,
width: 200.0,
color: Colors.red,
),
Positioned(
right: 0.0,
child: Container(
height: 250.0,
width: 150.0,
color: Colors.green,
),
),
],
),
),
),
| 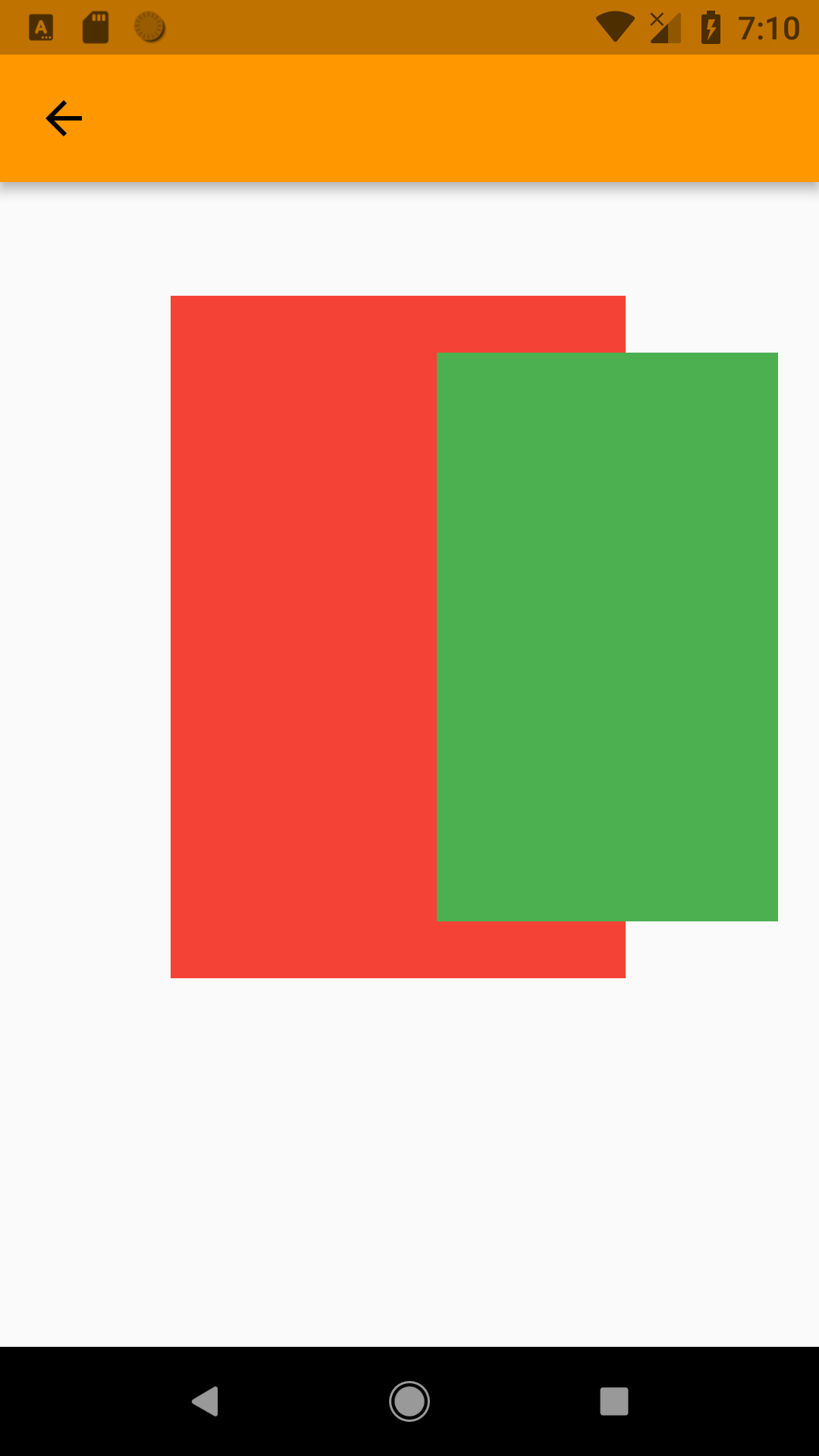 |